compoundbutton(Understanding the Functionality of CompoundButton)
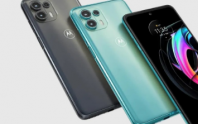
Understanding the Functionality of CompoundButton
CompoundButton is a user-interface element that allows developers to implement all sorts of toggle buttons on their Android applications. As the name suggests, it is a combination of two or more buttons. This button is well known for its intuitive design and easy-to-use functionality that allows users to toggle switches and perform various other functions. This article will provide a comprehensive overview of the CompoundButton, its various types, and how it can be implemented in your application.
Types of CompoundButton
There are several types of CompoundButton. The most commonly used ones are:
- CheckBox
- RadioButton
- ToggleButton
CheckBox: This type of CompoundButton is ideal for settings and preferences where users have to select multiple options. For instance, you can use it to allow your application users to select various categories of products they would like to buy. CheckBoxes can be set up to be checked by default, and users can either select or deselect the options based on their preferences.
RadioButton: RadioButton is a type of CompoundButton used mostly in forms and applications that require users to make choices from several options. Just like checkboxes, RadioButton can have initial values when the application is opened. However, only one item can be selected at a time on RadioButton. This attribute makes it ideal for use in polls, surveys and various types of authentication systems.
ToggleButton: ToggleButton is used when you want users to switch between two values. This type of CompoundButton can be set to on or off mode, and it is perfect for situations where you need users to make a choice between two exclusive options. For instance, you can use this button type to enable or disable notifications for your application.
Implementing CompoundButton In Your Application
Implementing a CompoundButton on your Android application is a straightforward process. First, you need to declare the CompoundButton in your application's layout file. After that, you can use Java programming language to implement the functionality of the button.
Step 1: Declare a CompoundButton in your XML layout file:
<CheckBox
android:id=\"@+id/myCheckbox\"
android:text=\"Buy product 1\"
android:layout_width=\"wrap_content\"
android:layout_height=\"wrap_content\" />
Step 2: Declare and fetch a reference to the CompoundButton in your Java code:
//Declare a class global variable
private CheckBox mCheckbox;
@Override
public void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.main);
//Fetch the CheckBox by id
mCheckBox = (CheckBox) findViewById(R.id.myCheckbox);
//Implement the setOnCheckedChangeListener of the CheckBox
mCheckBox.setOnCheckedChangeListener(new OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView,
boolean isChecked) {
//Perform some action here
}
});
}
Step 3: Implement the functionality of the button in onCheckedChanged:
mCheckBox.setOnCheckedChangeListener(new OnCheckedChangeListener() {
@Override
public void onCheckedChanged(CompoundButton buttonView,
boolean isChecked) {
if (isChecked) {
//Perform some action when it is checked
} else {
//Perform some action when it is unchecked
}
}
});
Conclusion
CompoundButton is a powerful and versatile user-interface element that allows developers to implement various types of toggle buttons in their Android applications. This article has provided an overview of CompoundButton types, and how it can be implemented in your application. It is important to note that CompoundButton can be customized to match the theme of your application, making your application more aesthetically pleasing and more user-friendly.
版权声明:本文版权归原作者所有,转载文章仅为传播更多信息之目的,如作者信息标记有误,请第一时间联系我们修改或删除,多谢。